In this article, you would learn:
- How to define basic routes in Laravel
- HTTP verbs at a glance
- Route handling, with and without controllers
- Route parameters
- Route names, and why you should use them
- Route groups.
It is assumed that you know basic PHP if you are reading this. It could well be your first Laravel article, but knowledge of PHP would be really handy.
Getting Started
A very basic and required feature of server-side frameworks is their ability to receive requests from users and return responses (these could be HTML pages or even JSON), these interactions are made possible with routes through HTTP(S), so you can guess how important routes are already.
Laravel makes it very easy to define these routes, point them to the code to execute, and solve many other routing needs.
By default, Laravel comes with a routes folder, to handle various routes needs, in its root directory, this folder contains four files, api.php (used to handle API routes), channels.php, console.php, and web.php (handles normal routes). In this article, our focus will mostly be on the web.php as that’s where all our routes will live.
You should have composer and Laravel installed to continue.
Let’s dive in
Create a new Laravel project, and head on to the web.php to get started. You should have the same content as I have here.
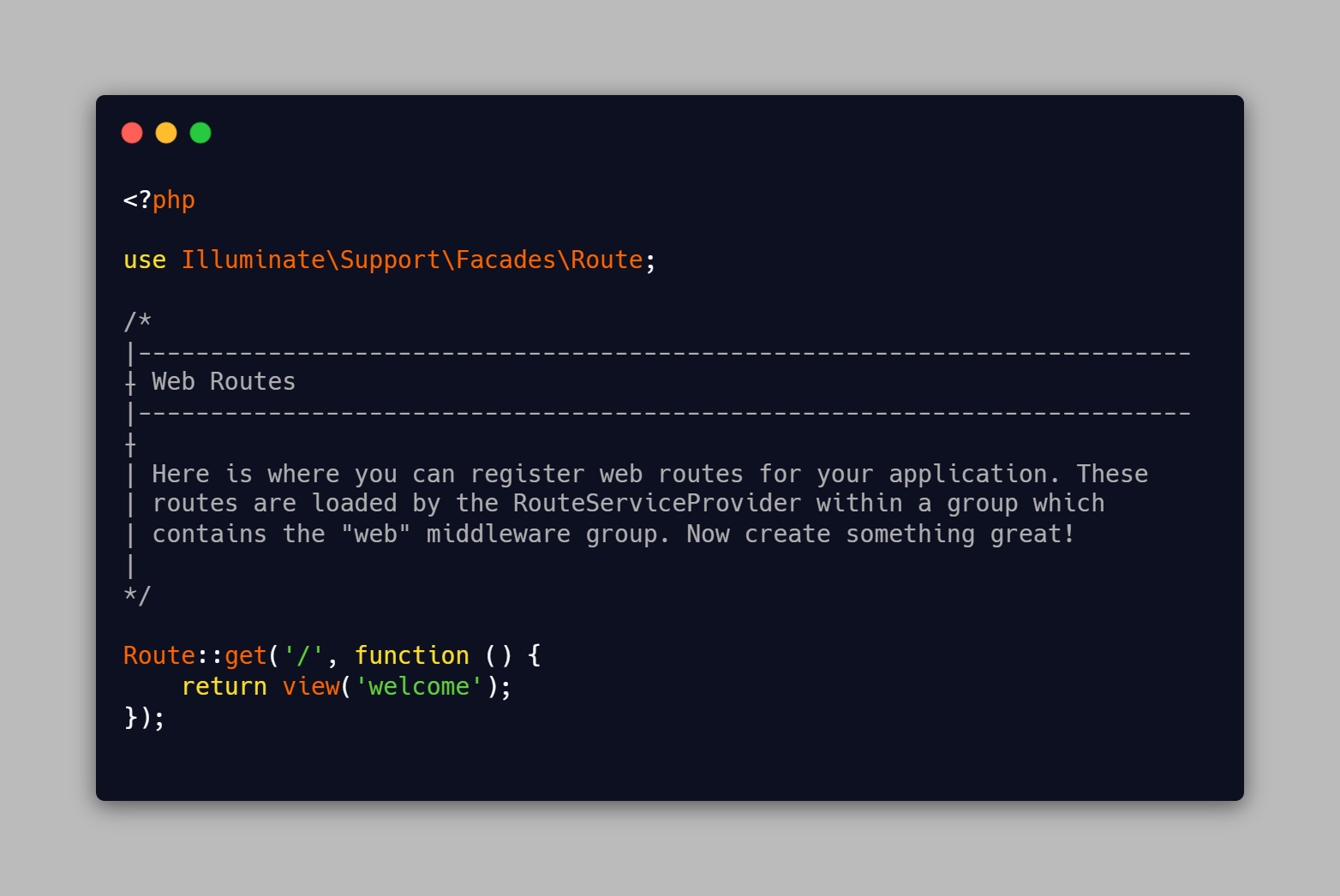
Here all we have is Laravel responding to the root route (of your domain), “/”, by using a closure that serves a welcome.blade.php file in our views folder.
Let’s have a break-down of what’s happening.
Route Definition In Laravel
Routes are defined in Laravel by using the Route class with an HTTP verb, the route to respond to, and a closure, or a controller method.
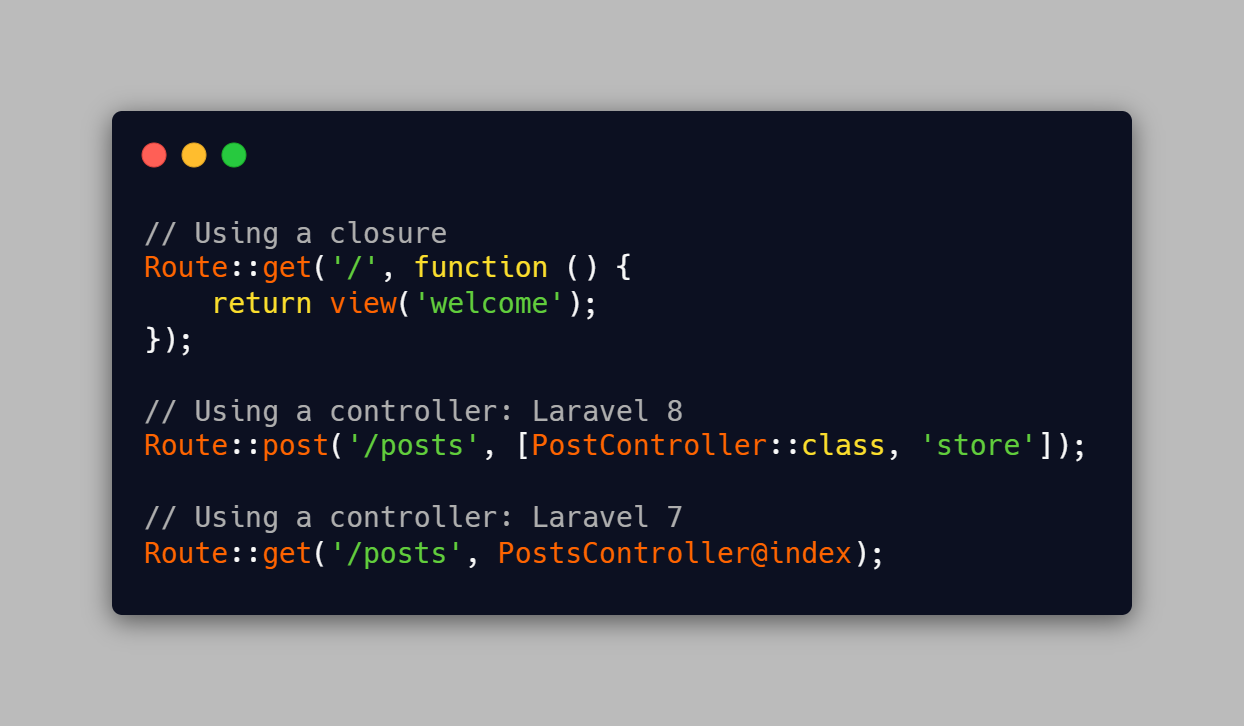
HTTP Verbs
HTTP verbs are actions that the HTTP request can use (actions users can perform). So in previous examples, we’ve seen the get and post verbs. Let’s take a closer look at what they do, and learn other verbs too.
GET: This is used mostly to fetch data from the server, without altering the data and returning it to the user.
POST: This lets us create new resources or data on the server, although it could be used just to send data, for further processing (this could be data validation, as in the login process). It’s considered safer than GET for sending sensitive data.
PUT: Put works like the POST in the sense that it lets you send data to the server, usually to update an existing resource instead of creating it. You’re basically putting it in. 😄
DELETE: The name kinda says it all, but let me explain. It removes an existing resource from the database.
Closures
A closure is basically an anonymous function, you don't have to name it to use it. They can be passed as objects, assigned to variables, and even be passed to other functions or methods.
You could build your entire application using closures but it’s only advisable to use them in very small applications, as it becomes quite messy (and even harder to manage) to have all of your application’s logic in one file.
Route Handling
With Closures
This is very easy and straight forward regardless of your Laravel version.
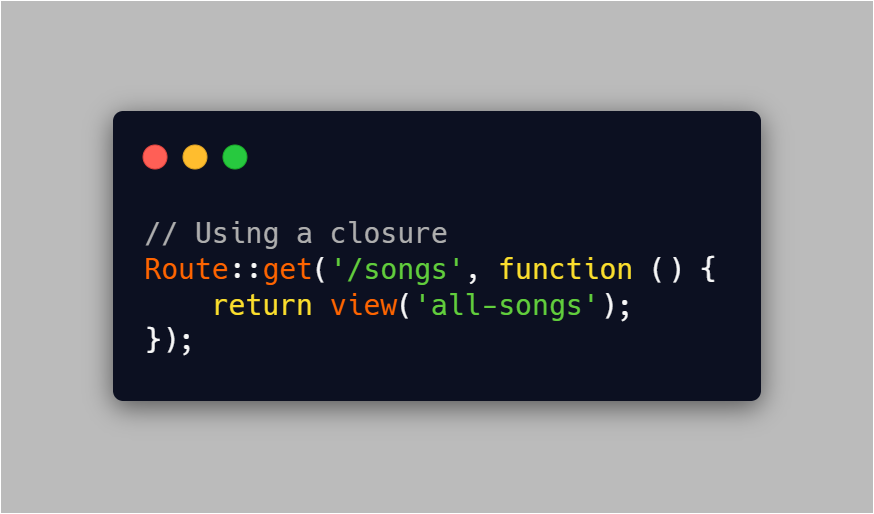
Notice the view() method used above, unlike other web development frameworks where you have to specify what folder contains user-facing views, Laravel has it all sorted for you and even provides an easy method for controller or closure — view relation.
So whenever you use the view() method, a blade file (.blade.php) or PHP file (.php) with the name in the views folder would be returned.
With Controllers: Laravel 7
This I would say is easier than the new update in Laravel 8, but you know it’s only my thought.
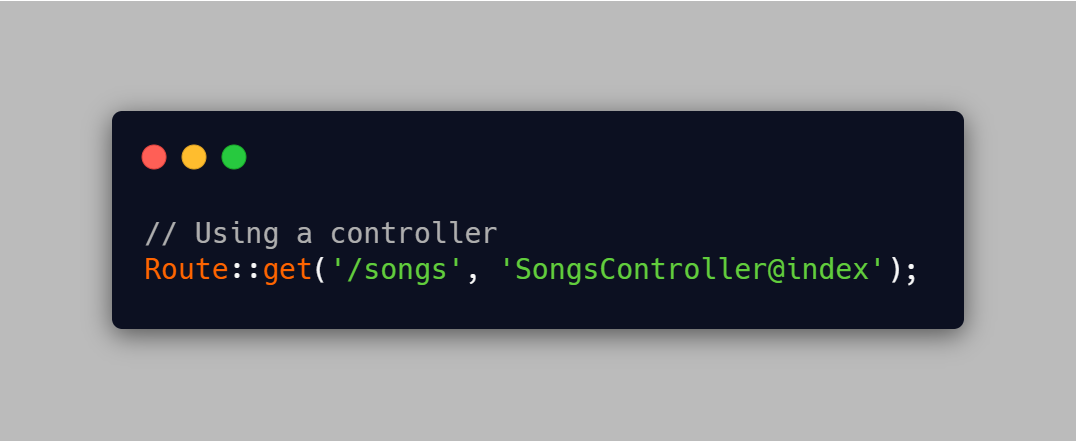
With Controllers: Laravel 8
With just some syntax changes, the idea of using controllers is the same as in Laravel 7.
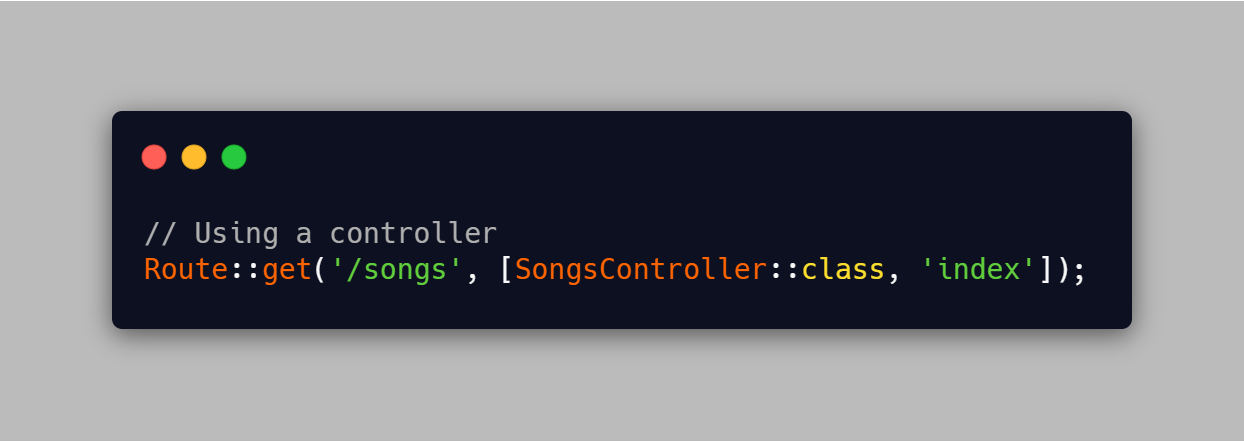
Now another difference apart from just turning the second parameter to an array is that you now have to import the controllers.
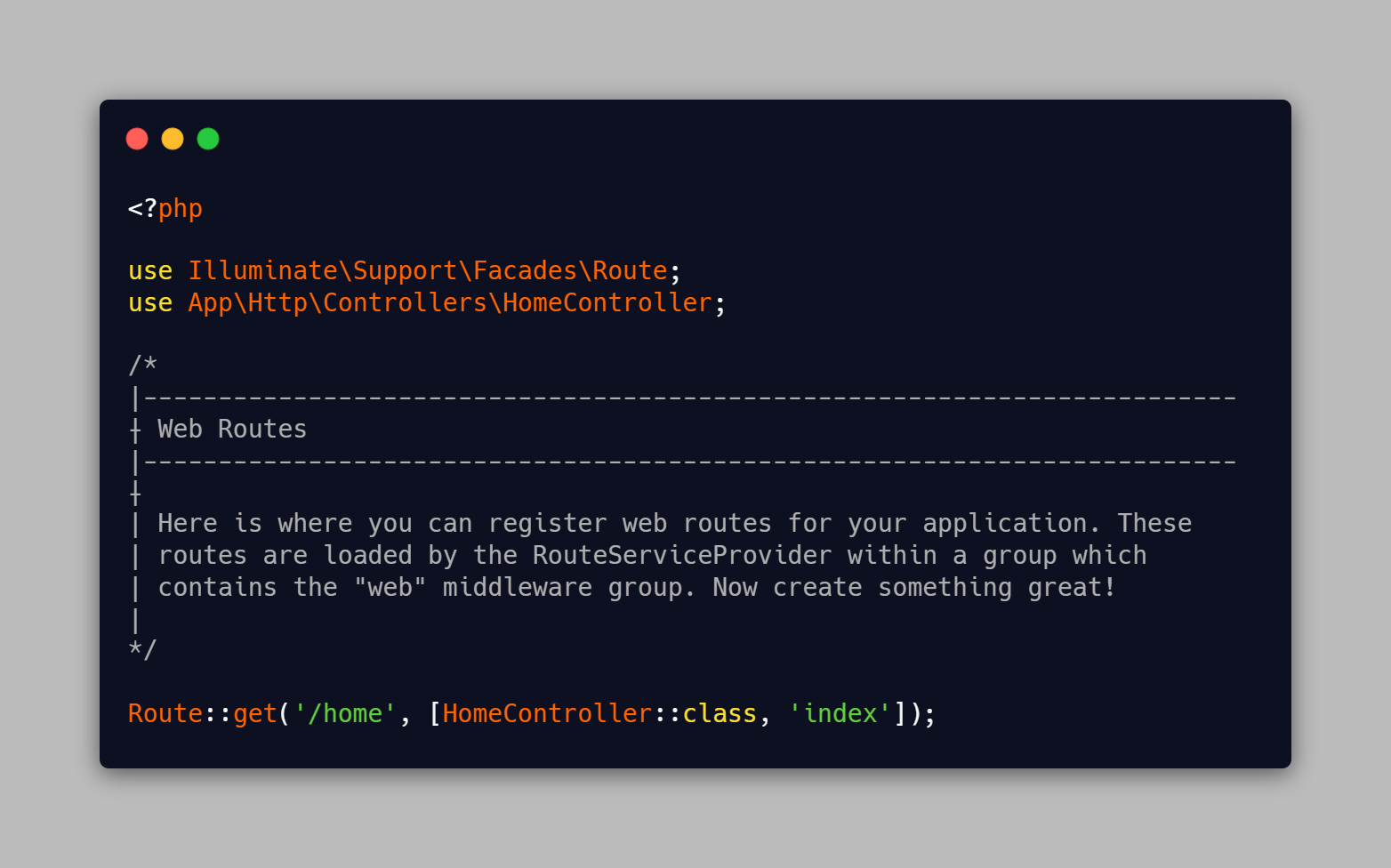
Route Parameters
Quite a lot of times, you need to have dynamic routes, to be used to serve a single page with dynamic content passed in the route.
Route parameters are segments in the URL structure that are dynamic.
We see route parameters every day, on every site, Facebook, Linkedin, Twitter, they all use dynamic routes.
Laravel lets you define dynamic routes very easily, with just some changes to the basic definition.
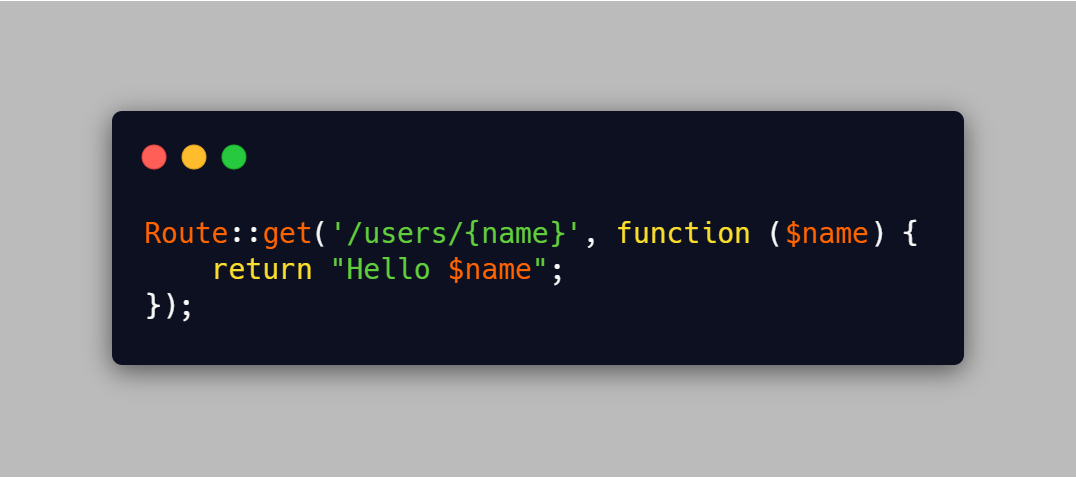
The “/users/” part is the static part of the route and the part in curly braces shows that it’s dynamic, visiting “/users/Zubair” would return “Hello Zubair”.
Notice how the variable was passed into the closure.
The route parameter doesn't have to always come last (at the end of the route).
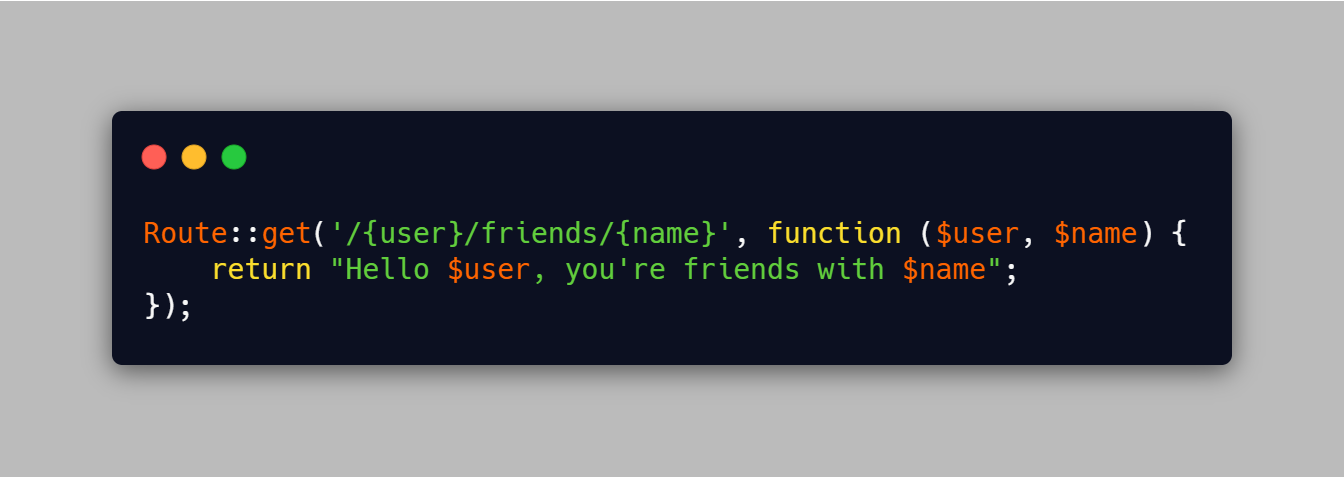
Route Parameters With Controllers
The route is still defined normally and the controller method doesn’t take much change too except that it is passed an argument (instead of being empty).
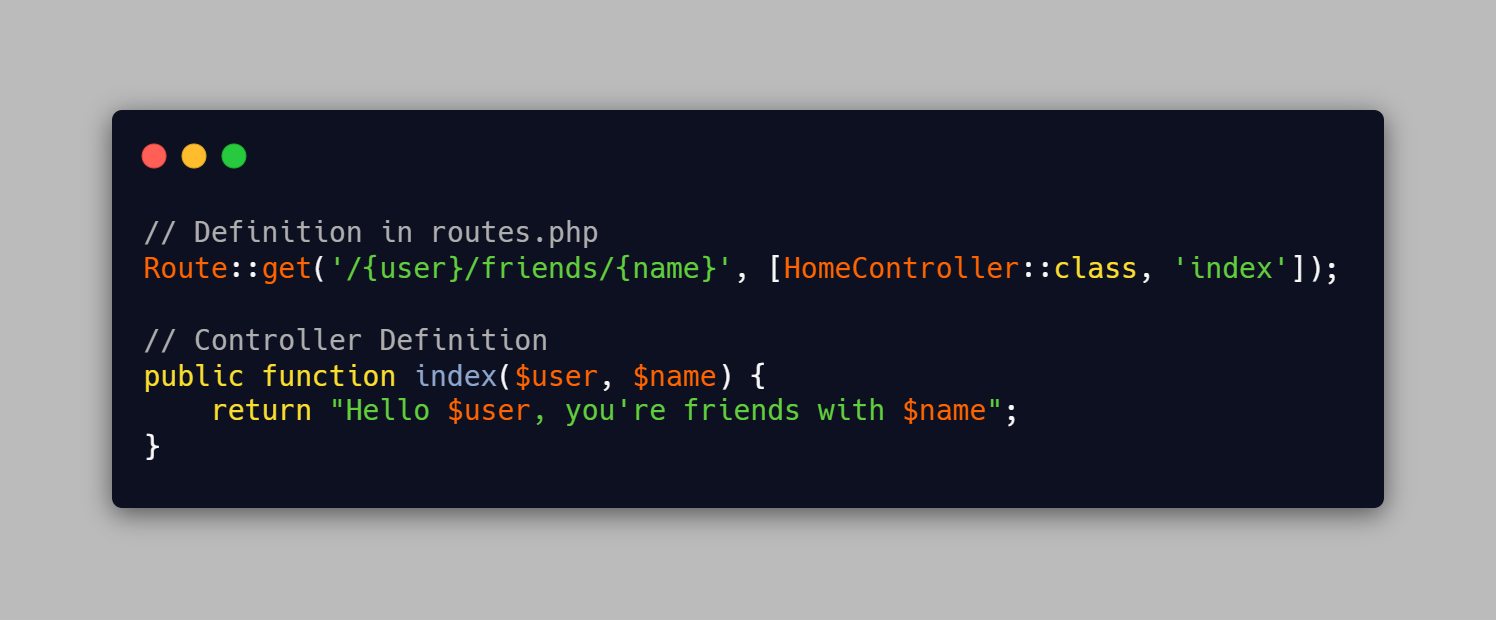
Don’t forget to import the controller in your web.php file
Route Names
Laravel provides a very simple and safe (maybe better) way of referencing your routes using simple names (like nicknames), instead of using long routes (the actual paths) everywhere (this could get messy and hard to maintain later on).
Each route defined in the web.php file can be named (a unique name saves a lot of issues) as shown in the example below.
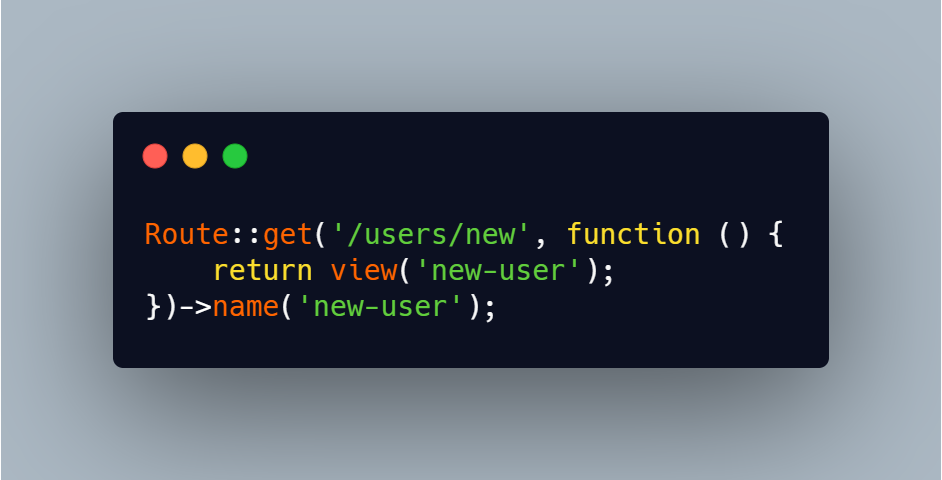
Why Use Named Routes?
Well, there are a couple of reasons, some of which are
- Sometimes, it’s shorter to write, users (a possible name for your users view) is better (maybe easily typed) than “/pages/users” which is the real route.
- Then, what I consider the greatest advantage is easy maintenance, having given a route a name, whenever you change the path without altering the name, the route is automatically changed wherever the name has been mentioned
route()
Laravel provides a route() helper to help reference the route by their names in your front end code.
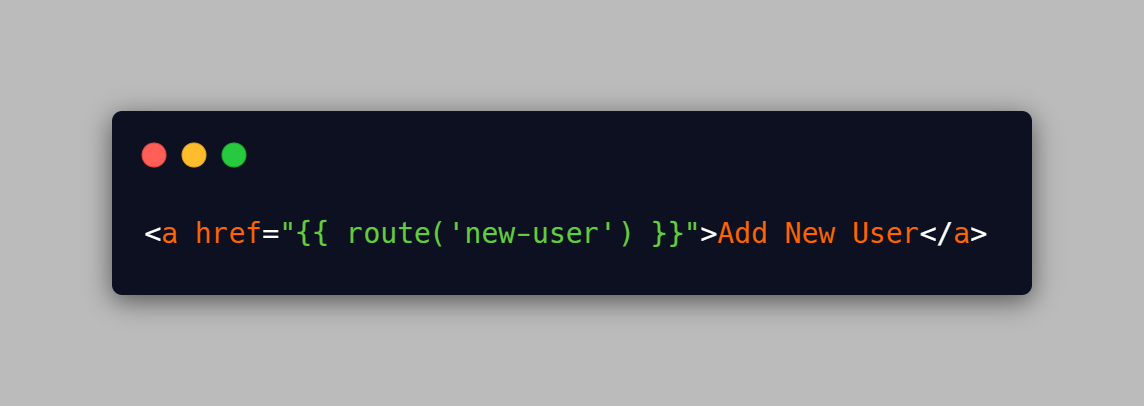
See how the route name was used instead of the path, so whatever change is made to the path now reflects here without having to make any other change.
Using Route Parameters With Named Routes
There’s really no difference in the route definition as all you’re doing is naming the existing route definition.
The real work is in the front-end (that’s where you use the route). Here you see how to pass parameters to the route() helper.
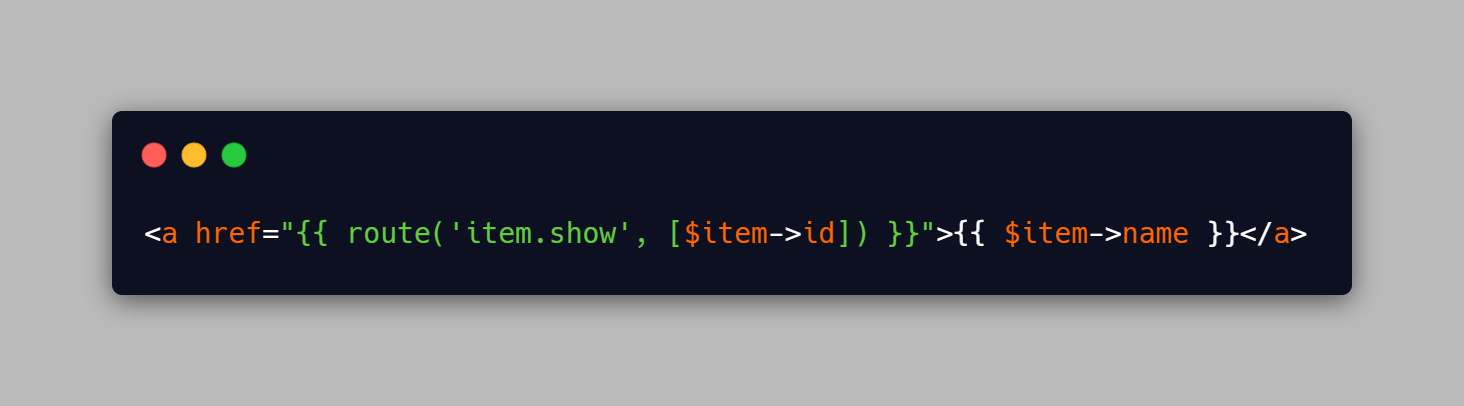
Suppose the method or closure accepts more than a parameter, you can pass them in many ways.
- You can order the parameters in the array in the route() helper the way they were arranged in the closure or method.
- You could make an associative array with the parameters as keys, and their corresponding values.
Using Query Strings With Named Routes
It’s also very simple (as things are always with Laravel) to pass query strings to named routes.
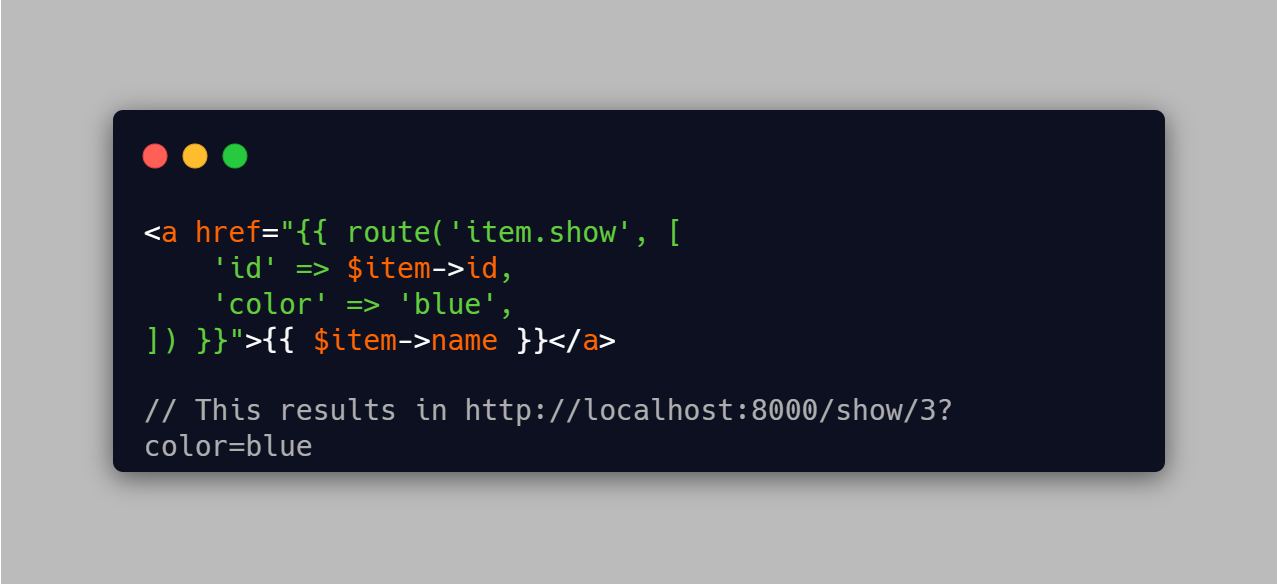
The query string is just passed as another element of the array, if there’s no corresponding parameter in the closure, it’s tacked on as a query string.
And I guess that’s all, follow me know when I release my next article on about route groups. Until then, do stay safe, and thanks a lot for your time reading this.
The End
If you have any questions or relevant great advice, please leave them in the comments section.
To read more of my articles or follow my works, you may connect with me on LinkedIn and Twitter. It’s quick, it’s easy, and it’s free.