After reading this article, you should know:
- What an array is,
- Various types of arrays
- Adding elements to an array
- Removing from an array,
- and still a lot.
To get started, you should know:
- Basic PHP, variables, and how to output to the screen.
- Having prior programming experience would help.
PHP is a server scripting language and a powerful tool for making dynamic and interactive Web pages.
PHP is a widely-used, free, and efficient alternative to competitors such as Microsoft’s ASP.
PHP 7 is the latest stable release.
Being a popular programming language, it allows for data manipulations with its many data types.
Getting Started
An array is a data structure that stores one or more similar type of values in a single value.
An array could be said to be a collection of data. It could be an empty collection like an empty box, or with just one item, like a car with just one person in it — it could take more you know, or with as many items as you want.
Arrays are of various types, numerically indexed arrays, associative arrays, multidimensional arrays.
Creating An Array
An array is created like any other data type in PHP, it is assigned to a variable.
An array can be created in many ways.
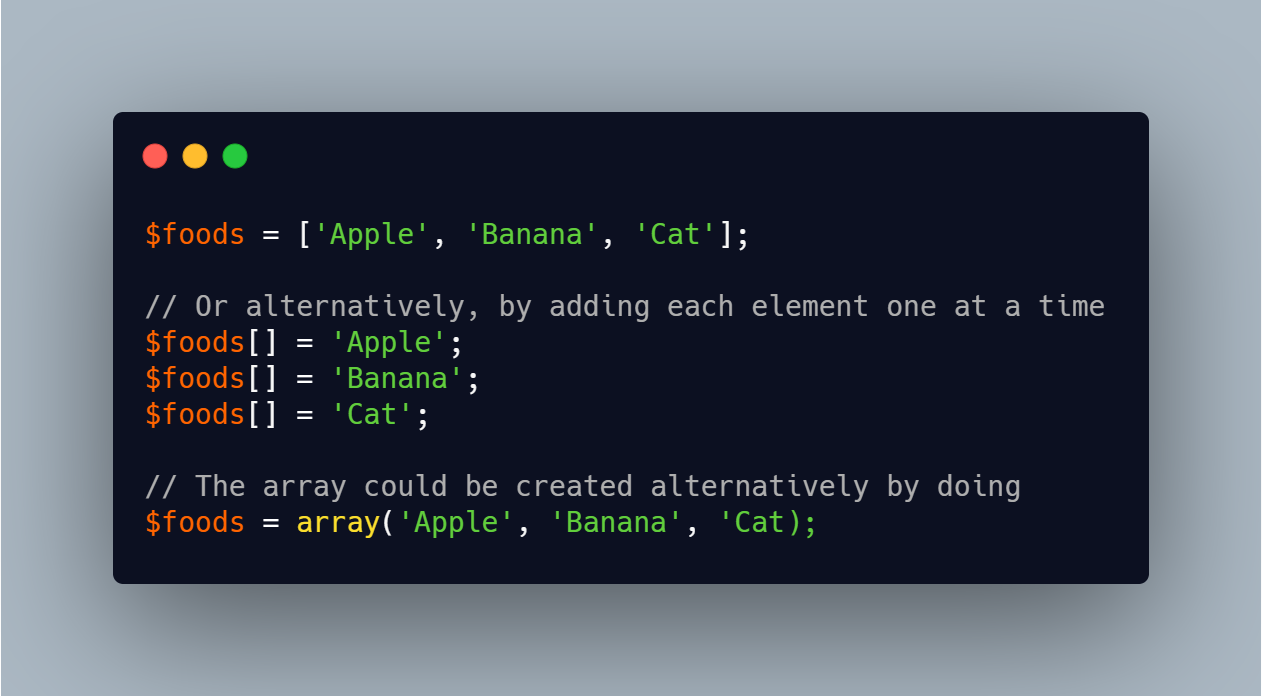
Other times you could want an array so simple yet too stressful to type. You could want an array of numbers or an array of English letters.
Range()
The range function helps autogenerate the content of the array given a starting and endpoint.
Printing The Array
So now we have our food array, it contains some foods — I know Cat is not a food, or is it? Let’s keep going. We want to output our array to the screen on our browser or terminal.
We use the _printr function, it displays information about a variable, in this context an array, in a way that’s readable by humans. Read more about _printr here.
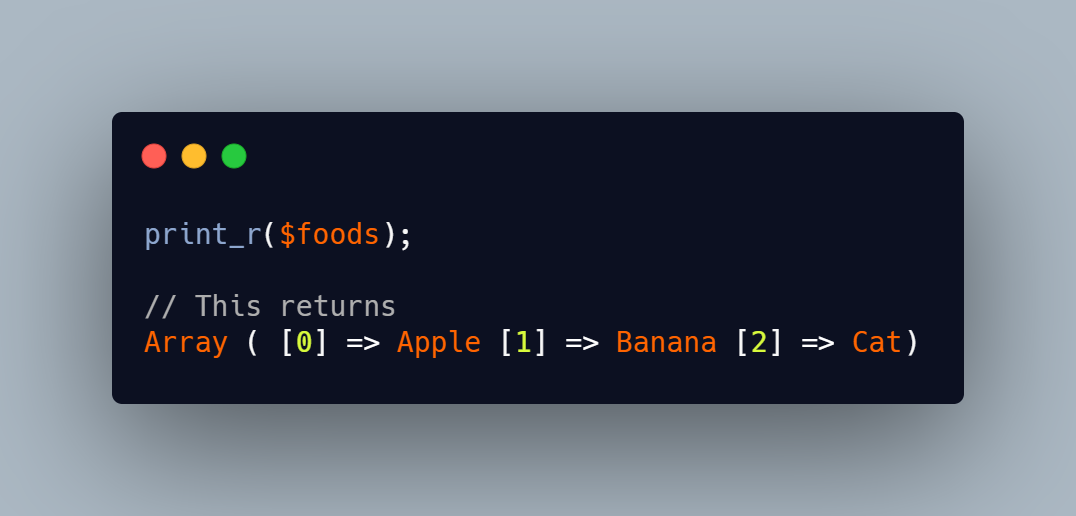
Now, you must wonder what the numbers in the array are, let’s see what they are in the next section.
Array Index
PHP arrays are made up of elements, each item in the array being regarded as an element, with each element having an index with which it can be referenced.
The indexes are auto-generated when you create the array, with the first element having an index of zero — PHP like other programming languages is zero-indexed but the index could be specified if you want.
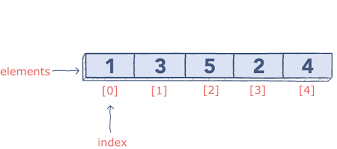
PHP Array | Educative.io
So, the first element in the array has an index of 0, the second an index of 1 and it continues in this pattern.
To specify your indexes, simply create the array this way:
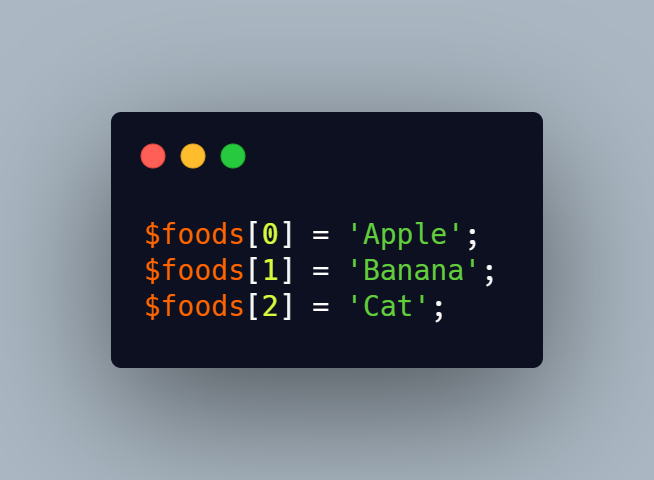
Now how do you print out only one item of your array? Keep calm and keep reading.
Printing An Array Element
So many times when you do not want to print out every element of your array, but only one.
You use the index to select the single item and echo it out.

Or you could even use it in a sentence like:
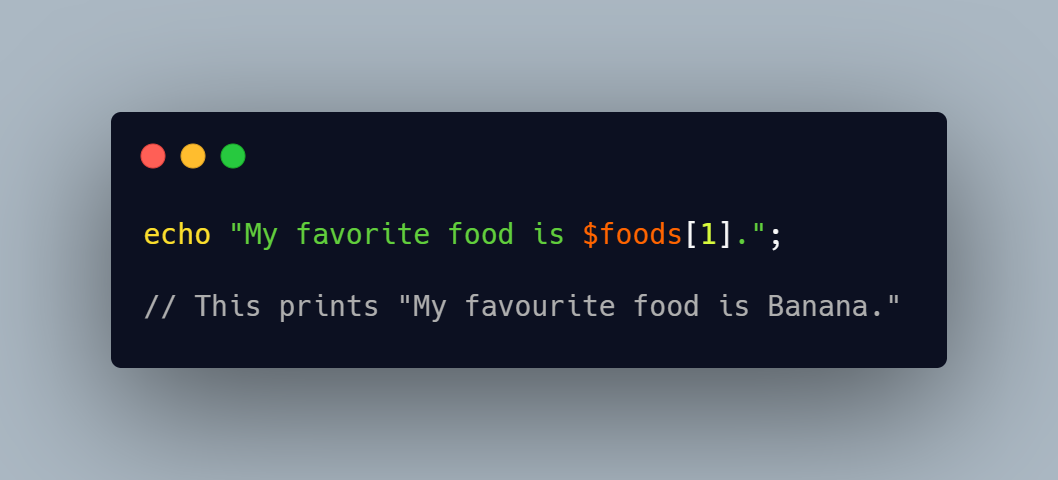
Other Array Functions
So in real-life applications, the array could grow large, that it could get hard to manage.
PHP gives us a bunch of functions to do that.
is_array()
PHP’s is_array() function tells whether the given variable is an array. It returns True if it is an array and False if not an array.
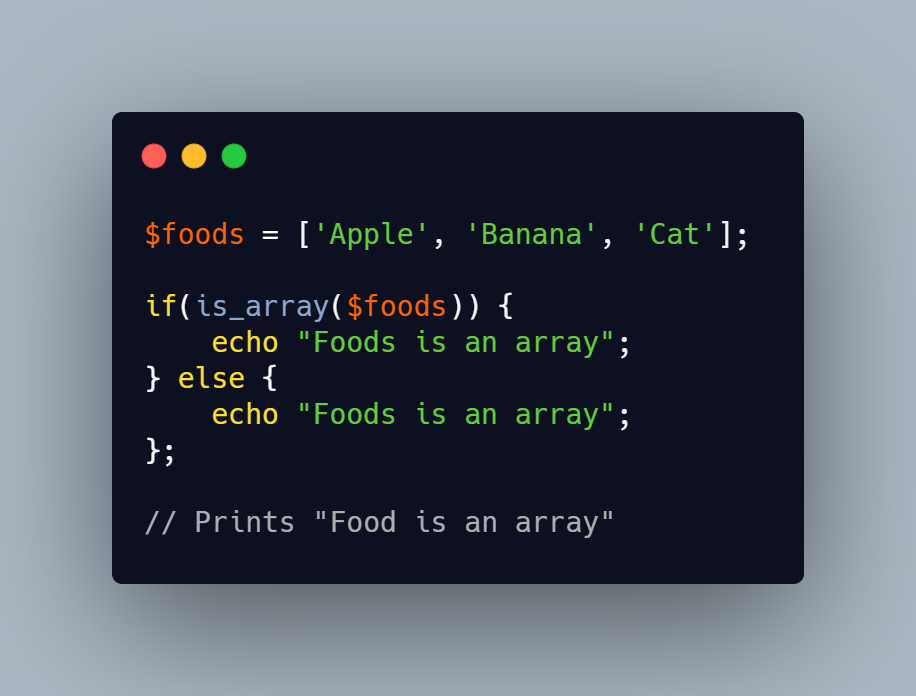
count()
PHP’s inbuilt count function tells us the number of elements any array has. This function is really helpful in deciding what to display in web apps. For example in a blog app, if the user has no posts or articles, display “No Articles Yet”, else display all the articles. So you use the count function to get the length.
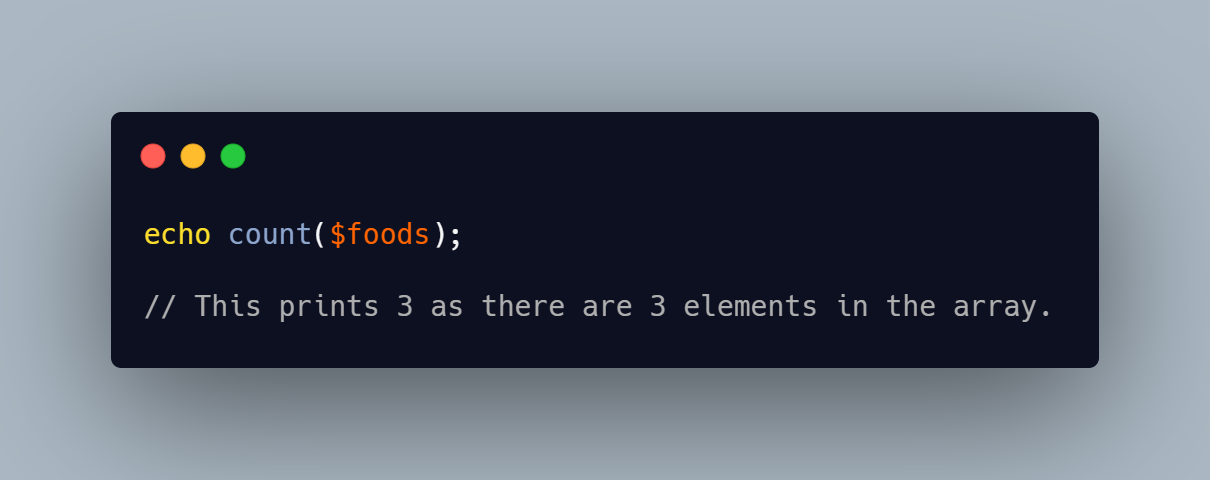
This function is the same as the len() function in Python or the length() method in JavaScript.
array_sum()
_arraysum() calculates the sum of all elements in the array.
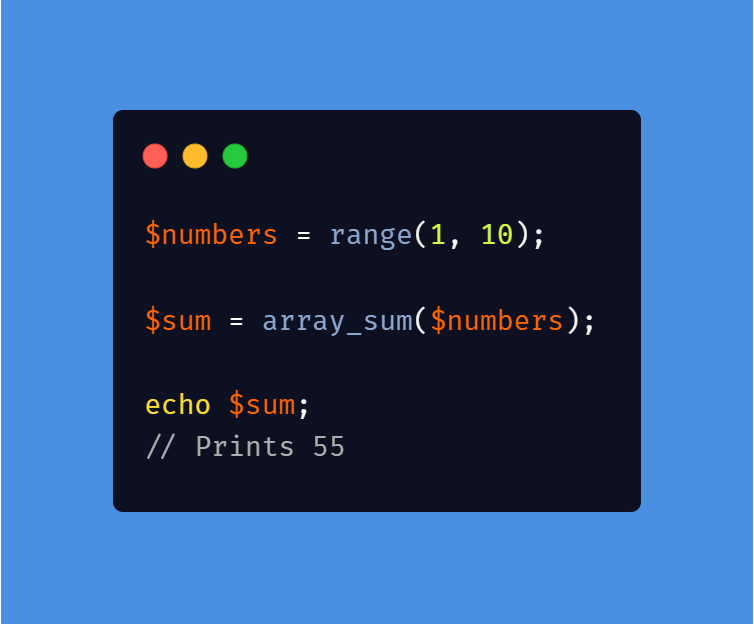
In this example, the array was generated automatically with numbers from 1 to 10, and the sum was calculated using the _arraysum() function.
sort()
If you want elements to be arranged from lowest to highest, then this function has is all you need as it returns the same array with its elements sorted.
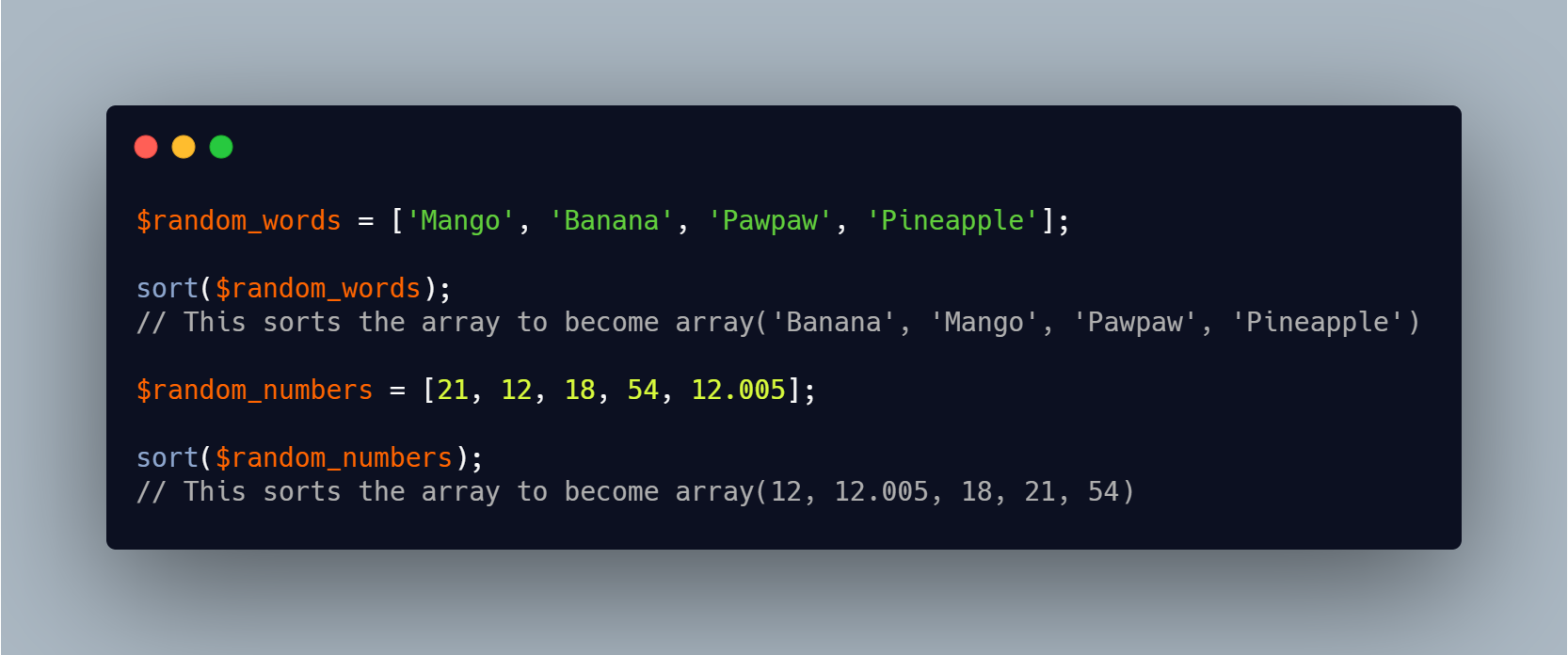
rsort()
This function does opposite of the sort() function as the values are not sorted from highest to lowest.
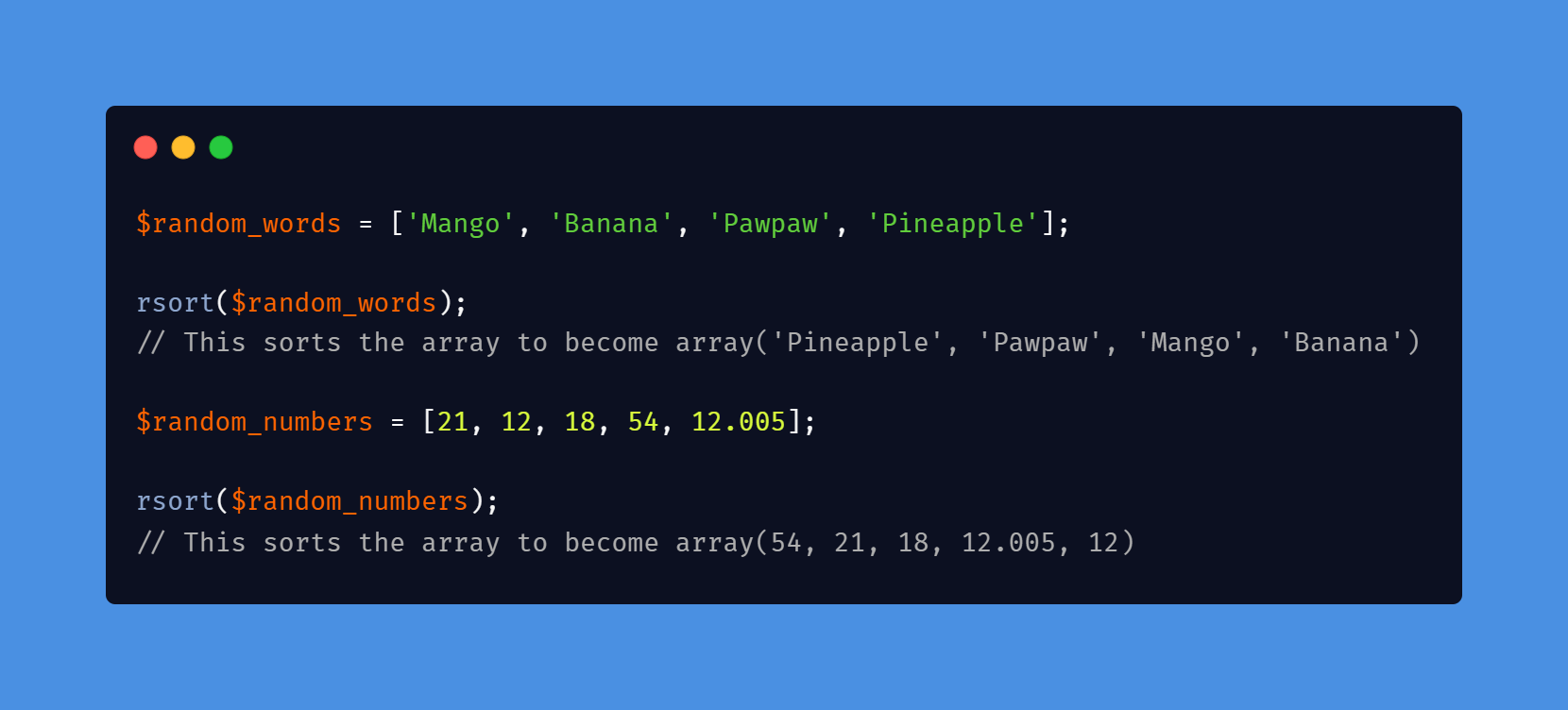
shuffle()
Some other times, you could want to build a random quote generator or want to randomize questions in an online test.
You use the shuffle() to rearrange the content of the array at random.
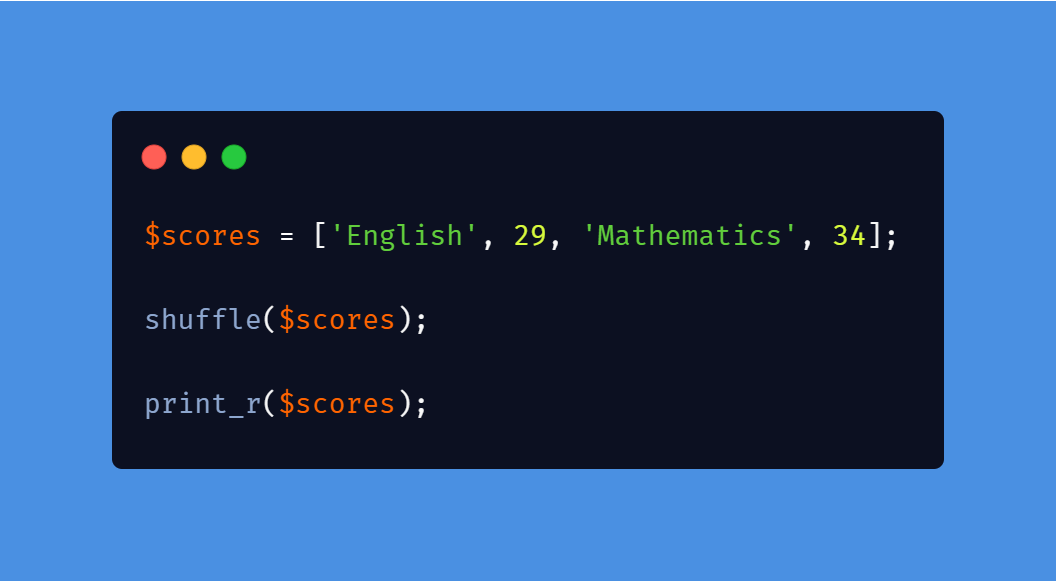
The array is returned with its content in a new randomized order.
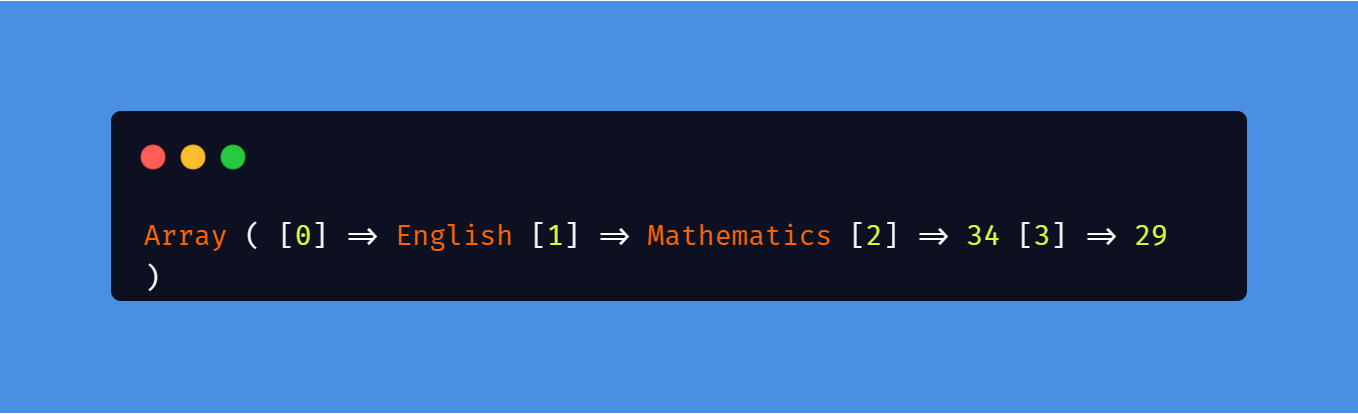
Note that on running this code, you could have an output that differs slightly from mine, this is because shuffle doesn’t rearrange in a specified manner. So running the code multiple times also will output different orders.
array_rand()
In some other cases, you just want a random value from an array, not shuffle the whole array, like in a game of dies, you want to output a random value between 1 and 6 every time the user plays.
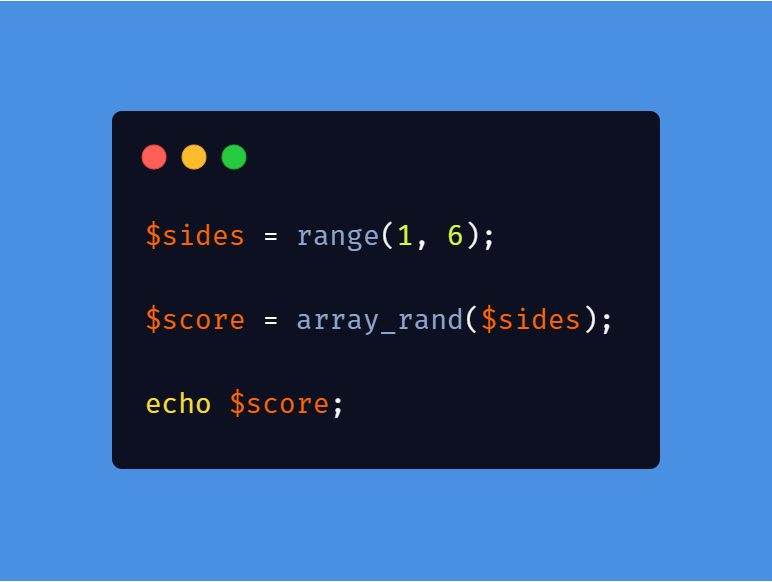
This code outputs a random number in the given range every time it is run.
in_array()
_inarray() helps you check if a given value exists in an array. It returns true if it exists and false if it does not exist.
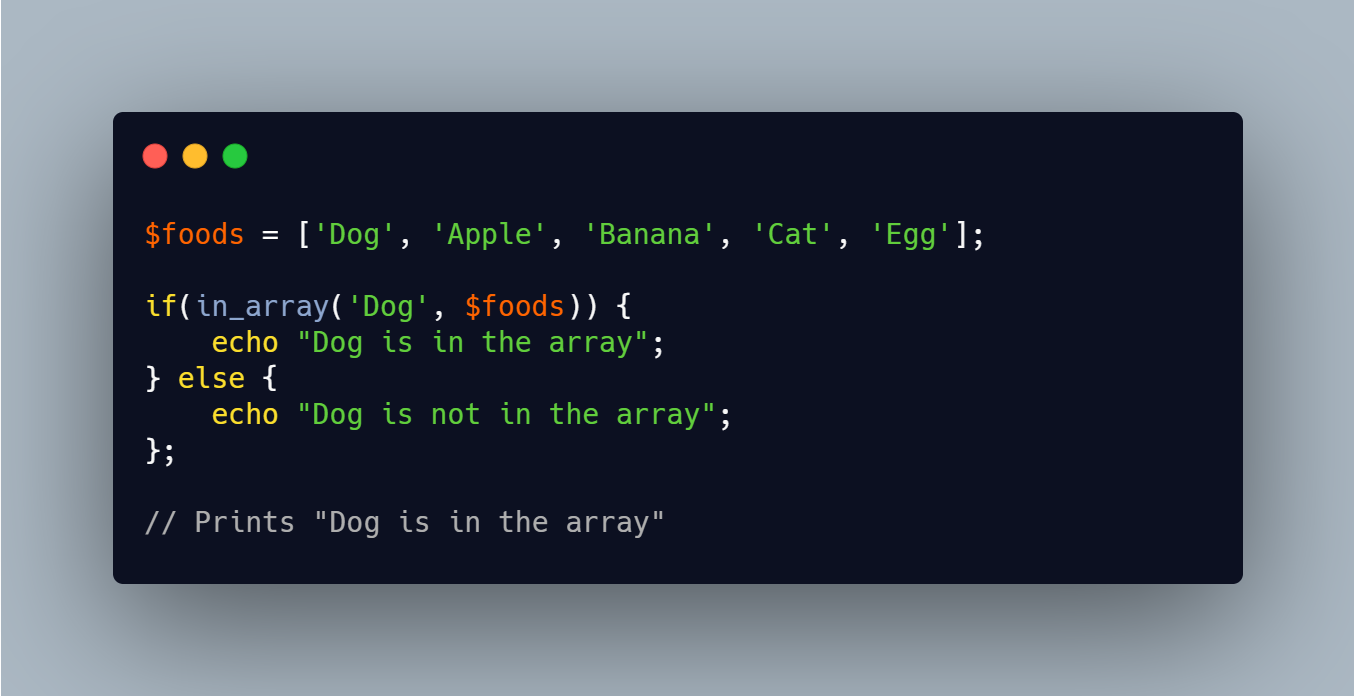
array_count_values()
So let’s say you want to make a report on what type of cars people love. So you decide to build a little web app where users get to enter their favorite type of car.
Now at the end of your data collection, you have a long array of cars. You use _array_countvalues() to know how many times every element of the array was repeated.
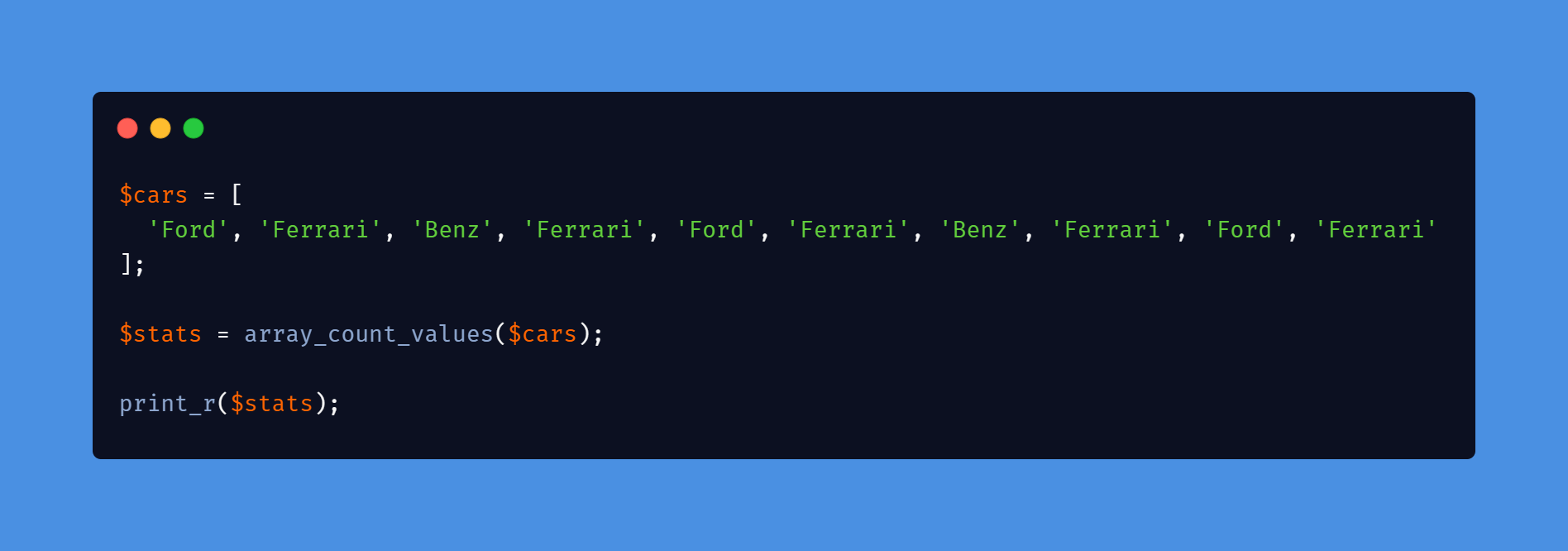
The array returned is an associative array with the elements of the given array as keys and their counts as values.

array_unique()
And some other times, you just want to know how many unique elements you have in your array. _arrayunique() returns an array of only the unique values.
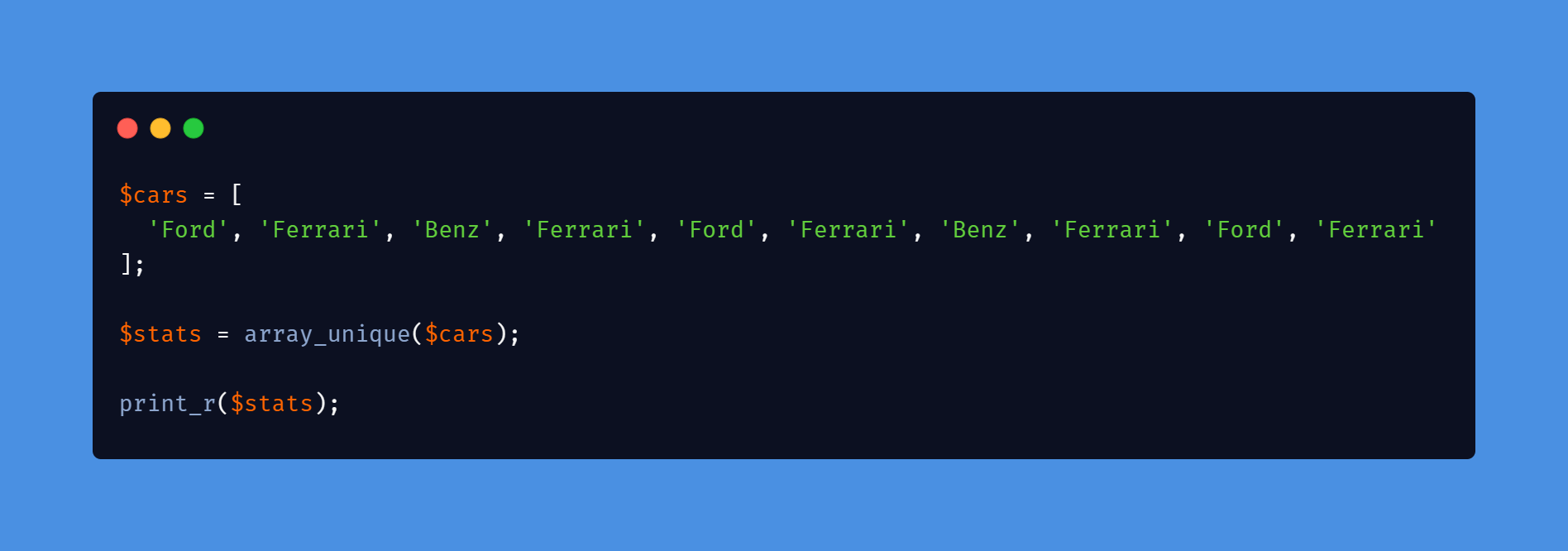
The returned array is:
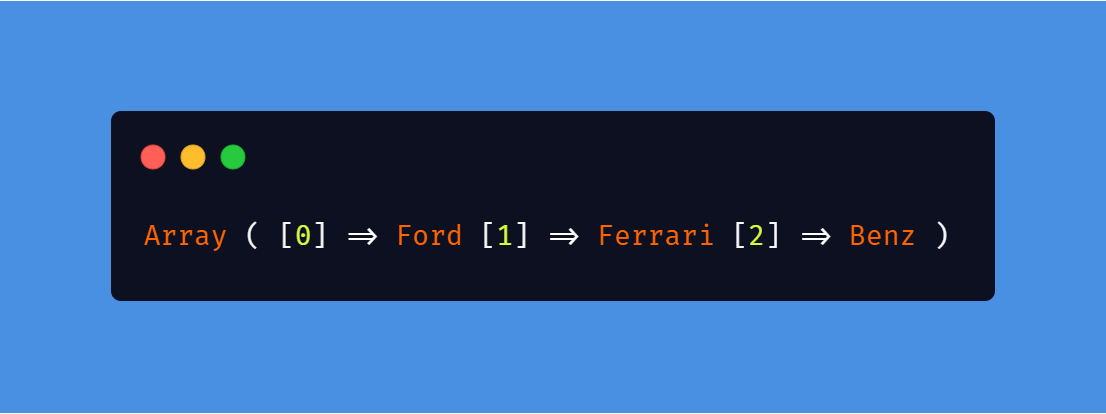
And it shows that you have only 3 different cars in an array with 10 elements.
array_diff()
Getting the difference between two or more arrays can be easily done with the _arraydiff() function, the function returns an array of values that are not common to the given arrays.

The returned array, in this case, has just an element as the only element in _fruitsa, not in _fruitsb is “Banana”.
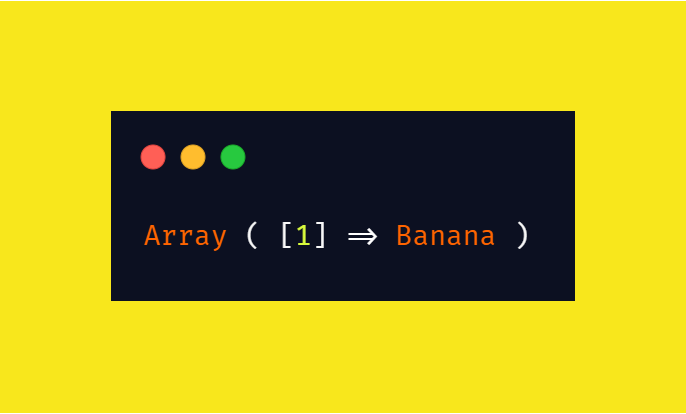
array_reverse()
_arrayreverse() returns the array with the elements arranged in reverse order without any sorting, just an order that opposes the order which they were before.

And so many other functions that will be discussed later.
Now, we can learn how to add elements to existing arrays. Elements could be added to the beginning of an array, to the end of the array, or a specific position in the array.
Adding To The Beginning Of An Array: array_unshift()
_arrayunshift() adds to the beginning of an array passed elements. The element or list of elements is prepended as a whole so that the elements stay in the same order. All indexes will be modified to start counting from zero.
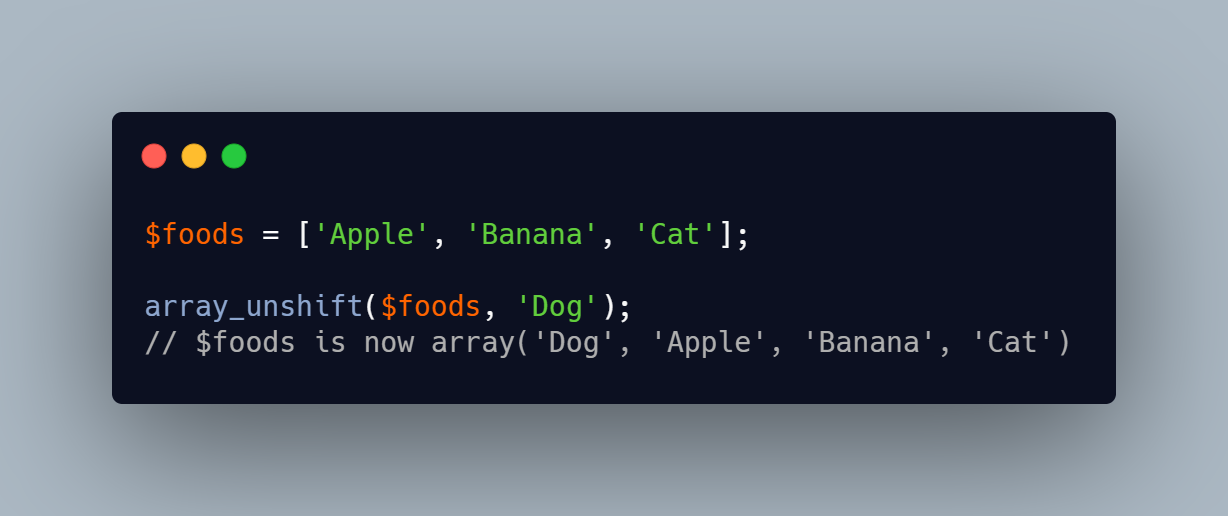
Adding To The End Of An Array: array_push()
_arraypush() treats the array as a stack and pushes the passed elements onto the end of the array. The length of the array increases by the number of elements pushed.
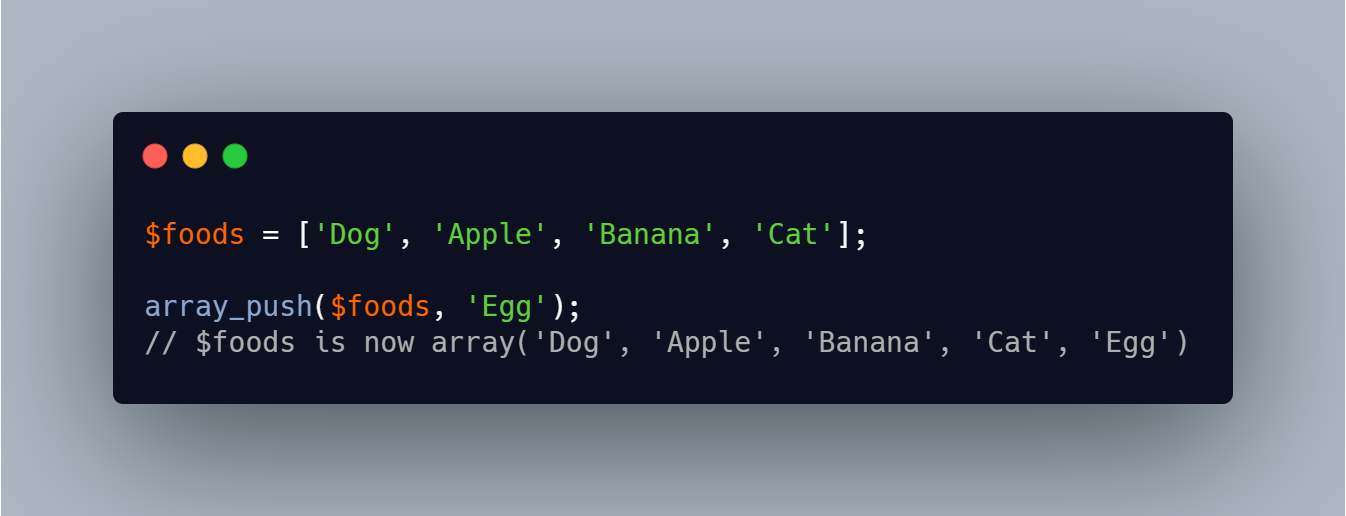
_arraypush() is especially useful when adding multiple elements to an array, but when adding a single element, its purpose can be achieved manually this way.

Alternative To Using array_push()
So far, we have only learned and worked with numerically indexed arrays, now let’s take a deeper dive into arrays by learning associative arrays and exploring many more array functions.
Associative Arrays
Arrays could go beyond using just numerical indexes to using user-defined keys or indexes.
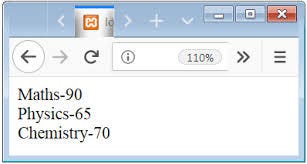
A use case of an associative array
So an associative array works like a dictionary with key-value pairs.
If we were to make an array of a student’s examination scores.

Creating an associative array isn’t too different from creating a normal array. So the other ways remain.
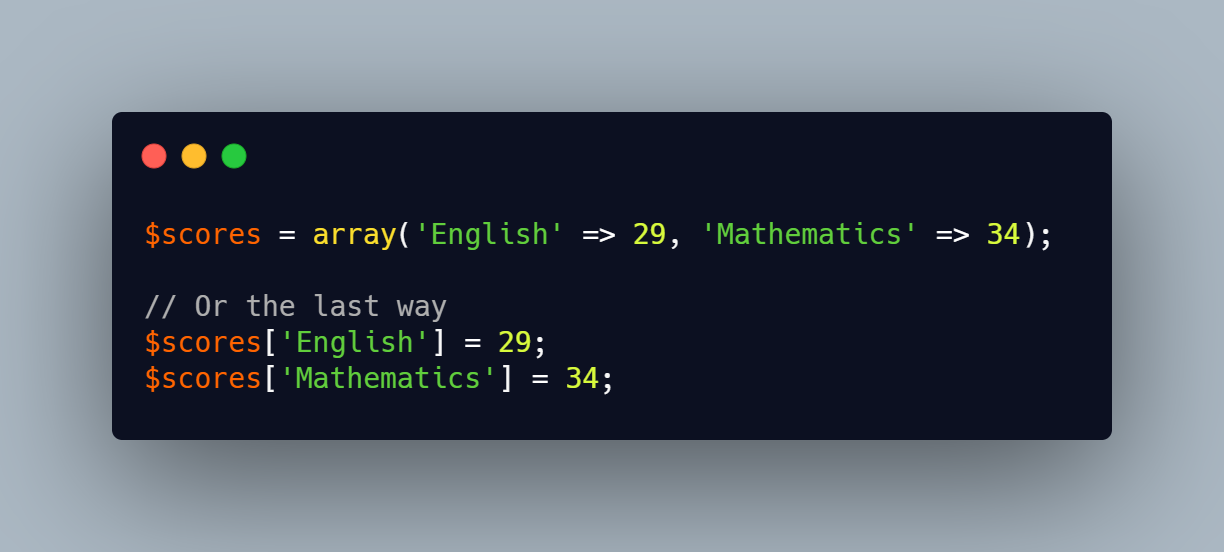
An associative array could be likened to a Python dictionary or a JavaScript object.
Printing From An Associative Array
Printing from an associative array is not too different from printing from a normal numerically indexed array as the only difference is that the keys are used to output instead of the indexes.

Using the values in sentences remains the same as using them in numerically indexed arrays.
Listing Out Only The Keys From An Associative Array
In the scores array we created above, we could need the subjects without the marks for some sort of evaluation or assessment.
We use _arraykeys() to get the keys only. It returns an array of the keys to us.

The array returned is numerically indexed and contains only the keys of the scores array.

Listing Out Only The Values From An Associative Array
Still using the scores array, we could get only the scores without having to get the subjects.
We use _arrayvalues() to get only the values. It returns an array of values to us.
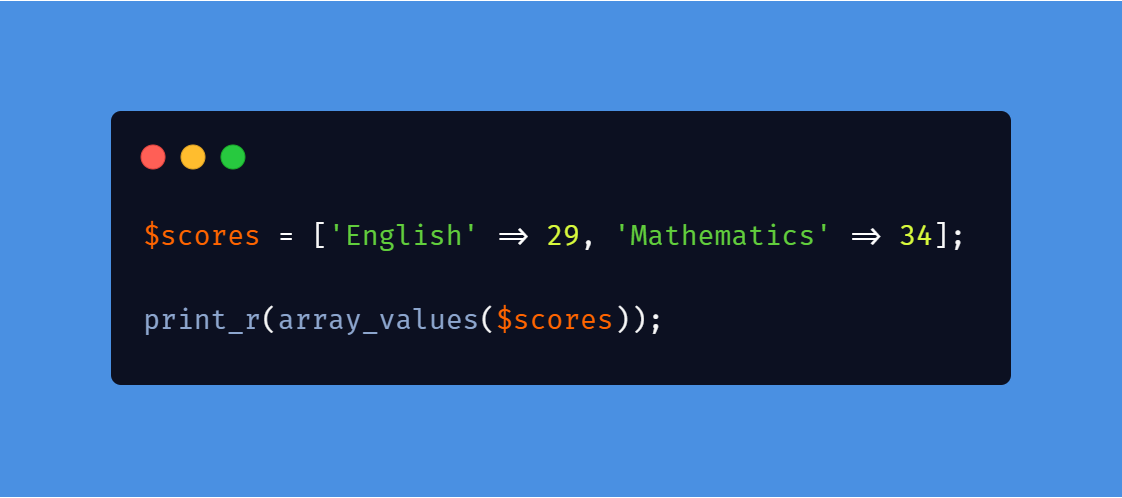
The array returned is numerically indexed and contains only the values of the scores array.
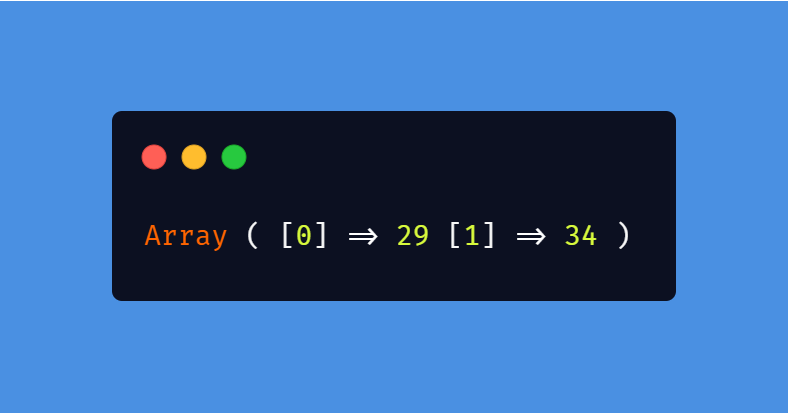
Checking If A Key Exists In An Associative Array
Still using the scores array, we could check if the student did a particular subject by checking for the existence of a key in the array.
_array_keyexists() will search for the key in the first dimension only meaning that this function works only for associative arrays, not multi-dimensional. It returns true if the given key is found in the array and false if it is not.
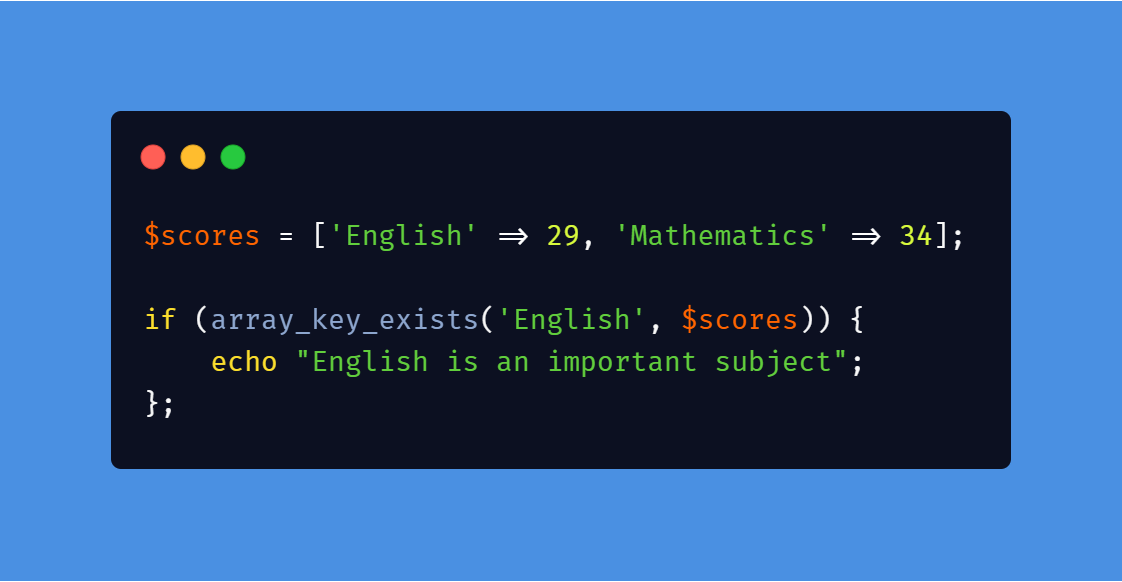
The code prints “English is an important subject” because the function returns true, as it can find “English” in the keys.
Arrays could get even more complex, in cases where you could need rows and columns. Then, you would need multi-dimensional arrays.
Multi-Dimensional Arrays
Multi-Dimensional arrays are basically arrays nested in arrays, where the outermost array could be seen as a table, and the arrays within it as rows, and then the elements of the array are the columns of your table.
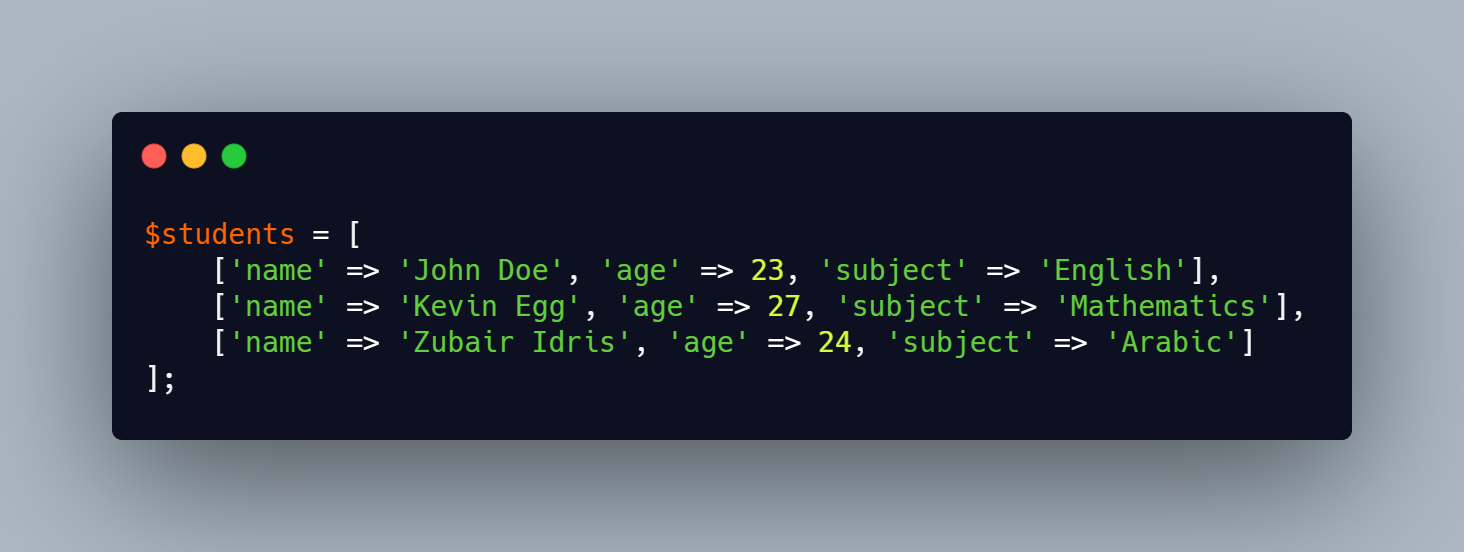
More Array Functions
array_chunk()
_arraychunk() splits an array into smaller chunks based on a given size. So the main array that’s being splitter becomes a multi-dimensional array as it now contains other arrays.

Running the code above gives the following result showing the smaller arrays.
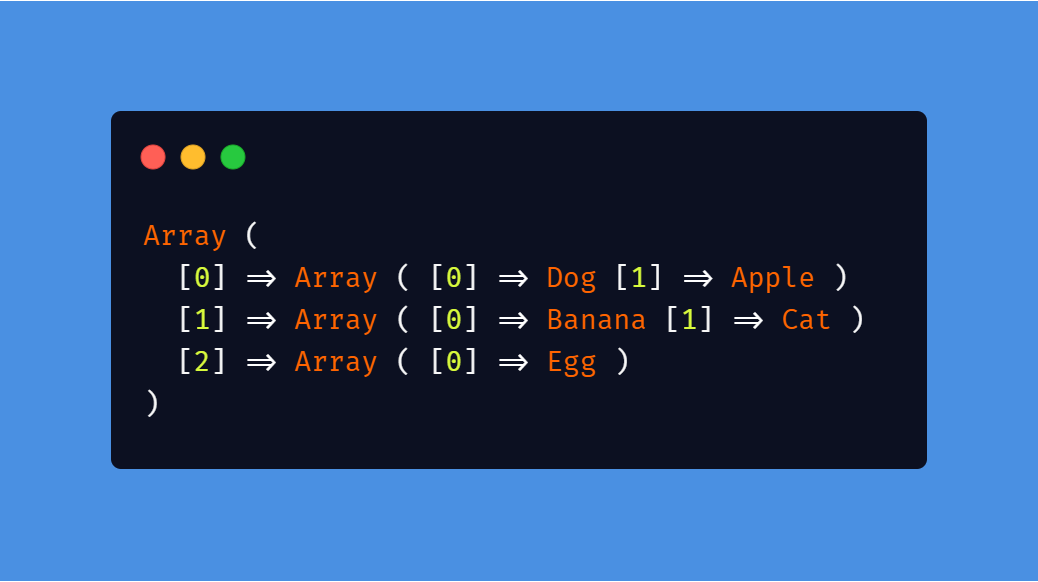
array_combine()
_arraycombine helps you create an associative array from two numerically indexed arrays using the elements of the first array as the keys of the newly formed associative array and the elements of the latter as the values of the array.
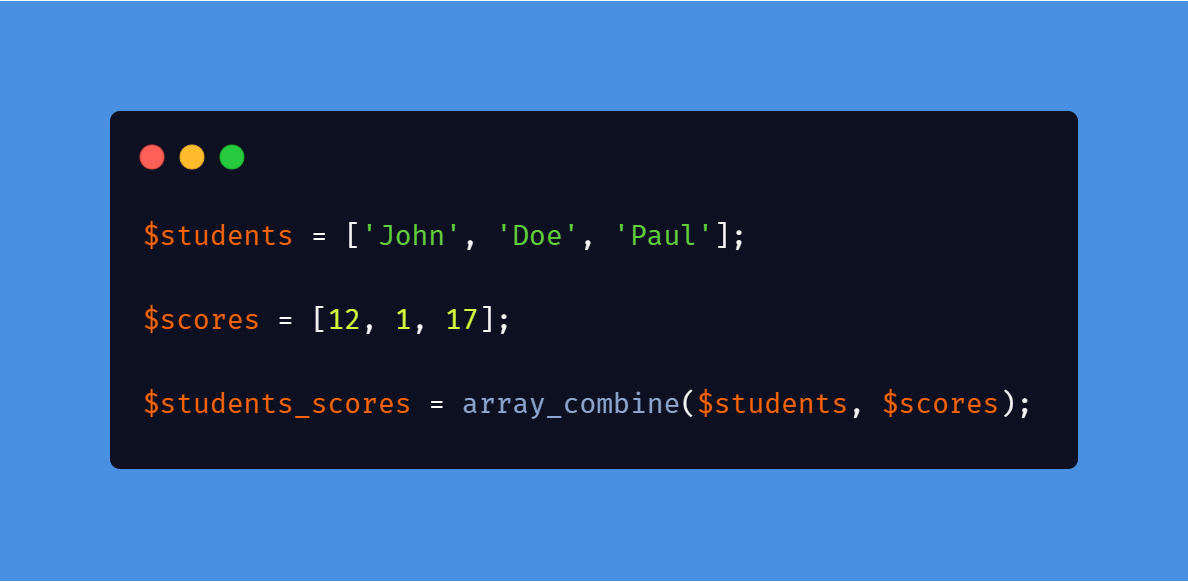
The newly created _studentsscores array is now an associative array and looks like:

array_merge()
_arraymerge() joins the elements of one or more arrays together so that the values of one array are appended to the end of the previous one. It returns the resulting array.
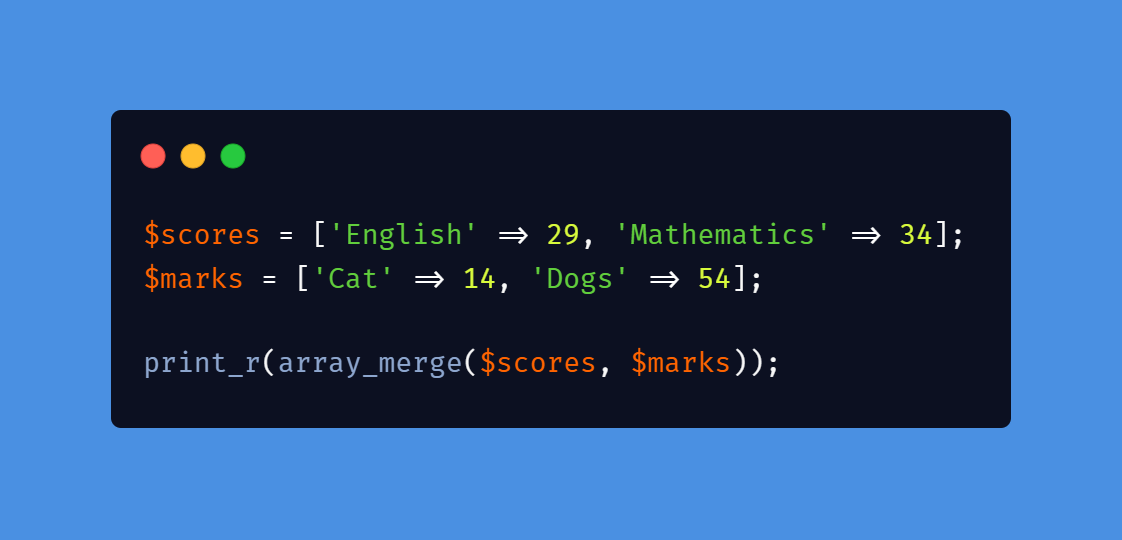
The code shown joins the scores array with the marks array, since they are both associative arrays and no key was repeated in both arrays, the resulting array has four key-value pairs.
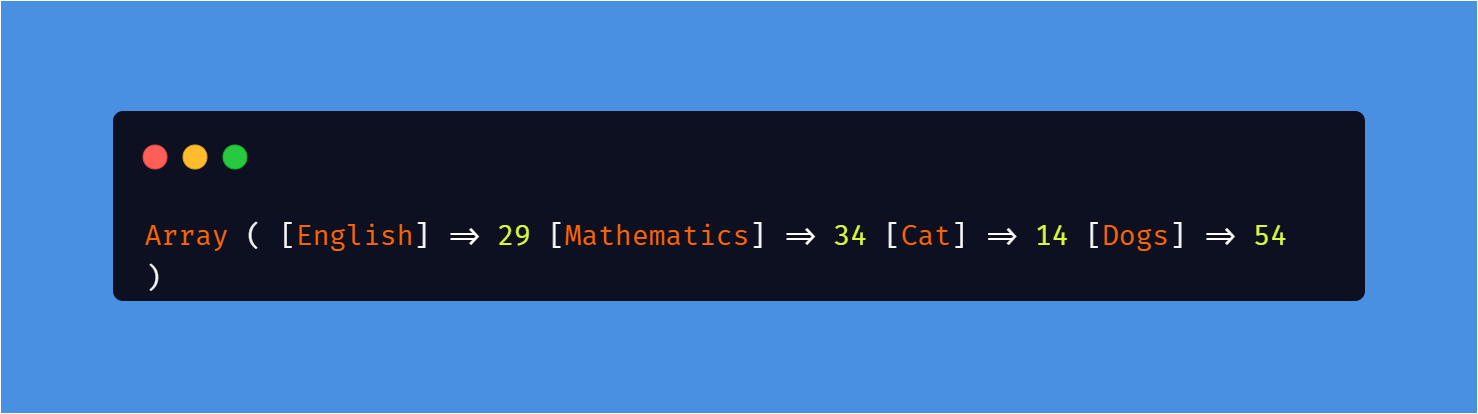
ksort()
ksort() sorts an array by key, maintaining key to data correlations. This is useful mainly for associative arrays.
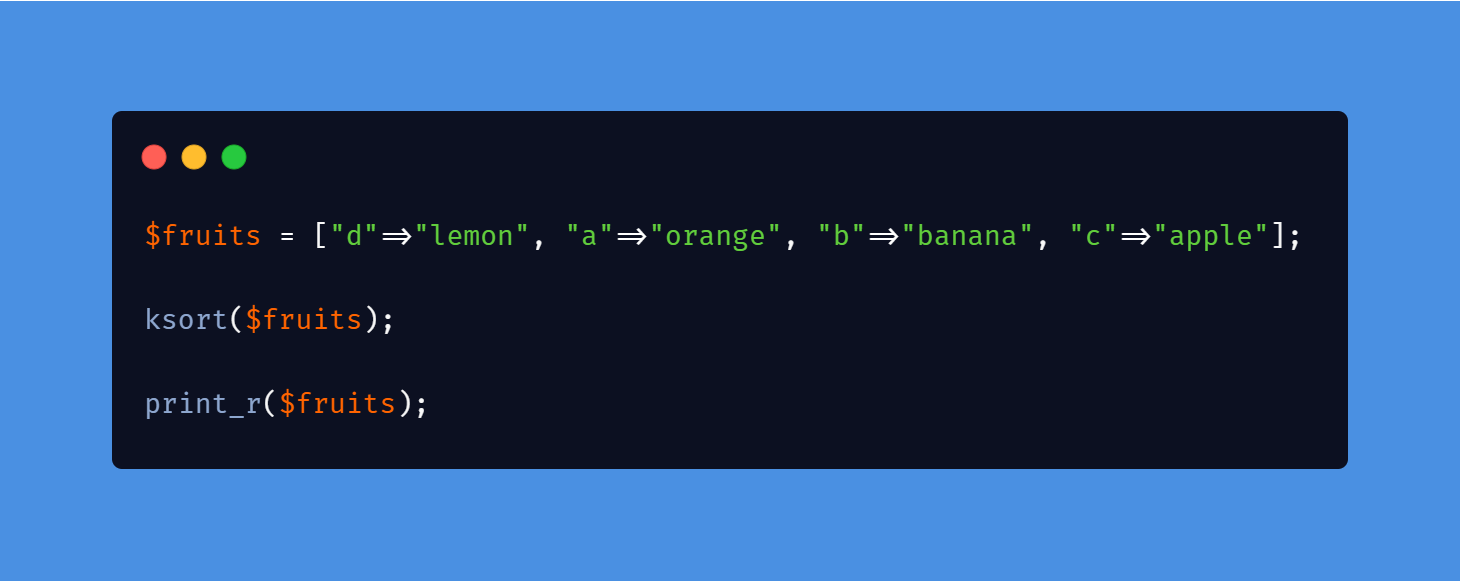
The returned array is now sorted by the keys.

array_diff_assoc()
Having seen how to find differences in multiple arrays with _arraydiff(), finding differences in arrays in associative arrays isn’t anyhow different too.
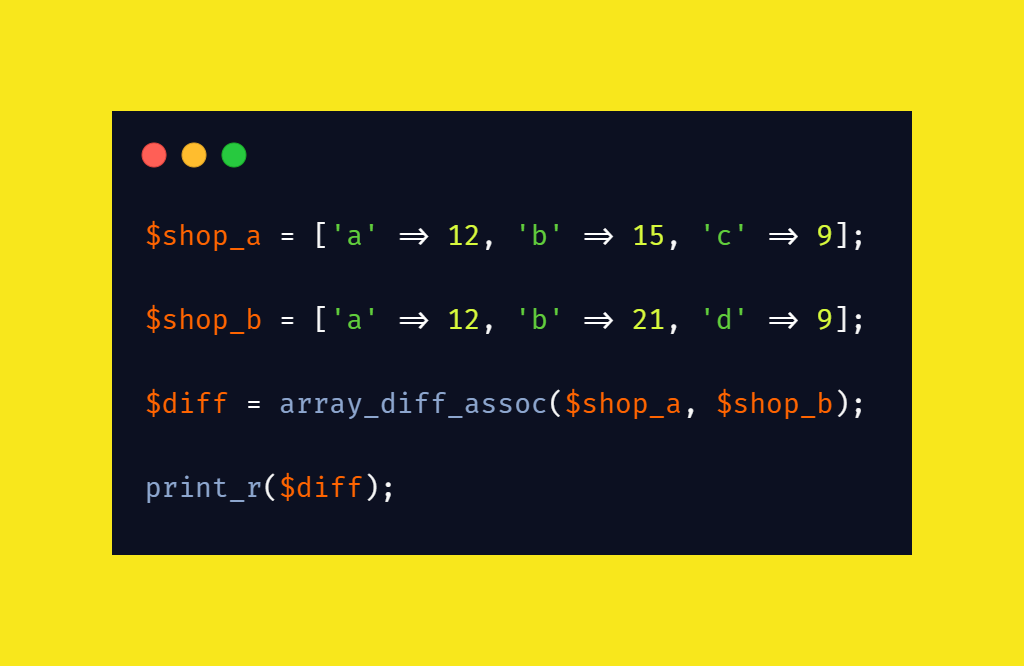
In the code above, the key “b” is present in both arrays but with different values and is thus regarded as different.
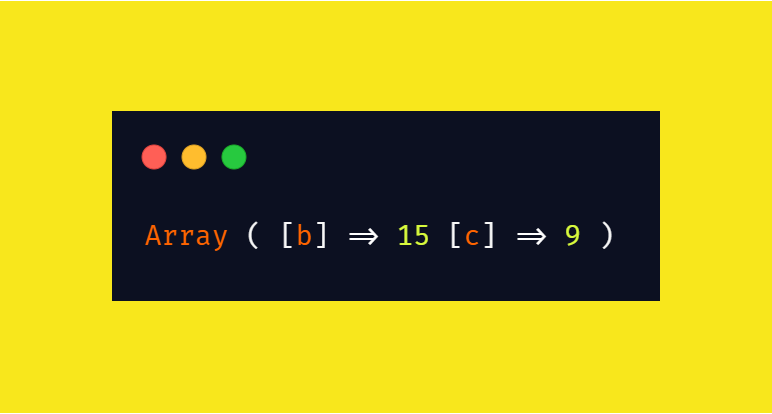
array_flip()
array_flip() helps you switch between key-value pairs in an associative array, turning the keys to values and the values to keys.

The returned array is still an associative array.
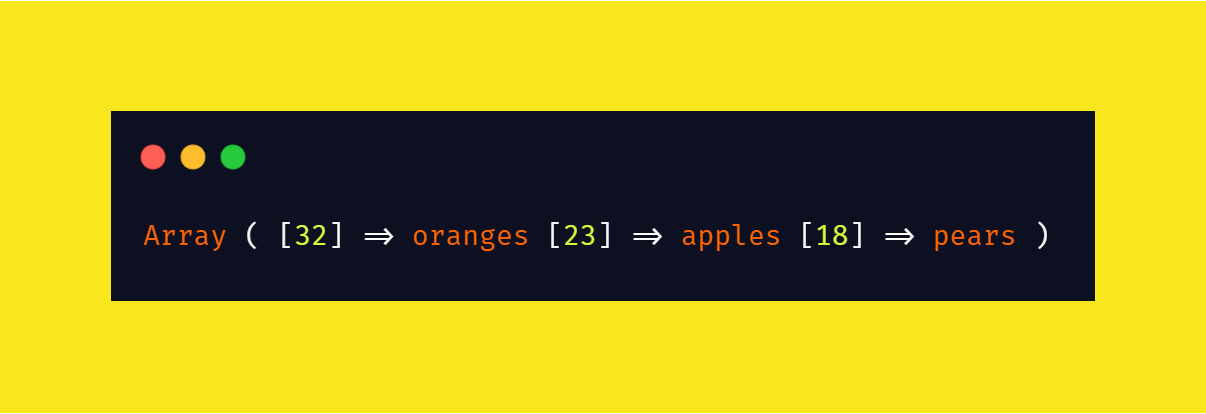
No matter what type of array was given to the function, the output is always an associative array. If a numerically indexed array is given, the elements become keys to their indexes.
At this point, you must have learned a lot about PHP arrays and must be quite comfortable working with them. Should you want to learn more, read the official documentation here.